Congrats on finding my website!
Anyway hi, I'm a linux hackerman in his early 20s who likes to code too many things at once.
btw I screwed around with the colour scheme, hopefully it's not too painful
Kovarust is my attempt at a free Minecraft clone. I'm aiming for it to be a viable replacement for Java Edition eventually. It's written in Rust, and will hopefully be faster and more versatile than the original Minecraft, with as many features actually implemented as plugins as I could manage.
I've redesigned every aspect of this thing about 5 times before it's even compiling, so it'll taking me a while to get it right, but it is progressing.
Tetris
Back in the good 'ol days before I started learning Rust (and subsequently rendered my PinePhone useless by desoldering its SIM card reader using rustc), I just felt like playing Tetris.
So, in between pretending I was learning how to do loops in java for
uni, I ended up making this in a day in C. It's under 400 lines long, so
it could be used to dissect and learn programming in C, I guess.
Not that it's especially good code, but still.
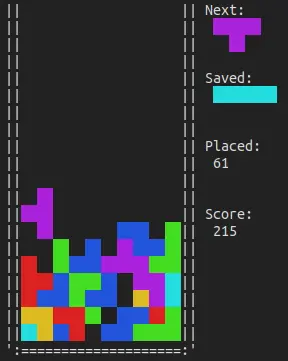
Random scripts
Just a collection of utilities/games I wrote in zsh for one
reason or another. Note you'll probably have to chmod +x
to
run these. Also I haven't tested them in bash becuase it's gay
and you should be using zsh.
bytecalc is a byte unit converter, that is, working in
multiples of 1024.
(fend is good for
this and a lot more)
list is a (janky) list
iterator - basically, loop over each line in a list and run a command on
it. If the command works, remove the line, otherwise keep it to maybe
retry later. I use it for mass curling memes and ignoring the
URLs with duplicate filenames until after I categorize the previous
batch.
NO MEME LEFT BEHIND
dewit is like a user-level version of runit (i.e. a user-service manager) that you can put in your crontab. I use it to run my website.
sc's just my screenshot
command, using crud to select a window/area and crud
to actually get the picture. Then it either copies it to the clipboard
or saves it to a file, if one is specified.
I had shotgun & crud listed the
wrong way for the longest time. -_-
pong.sh is Pong in shell. W/S & J/K to move the paddles. Good luck with the collision system.
1248.sh is a minimal shell implementation of the classic 2048, but in a terminal and with Vim controls (also featuring saving/loading games). To save on space, tiles are incremented linearly instead of doubling (2048 = 2 ^ 11). If you have any other questions, consult the sauce.
2D Raycaster
Back when I was early into learning C, I figured I'd try making a 3D-esque game engine similar to DOOM. I knew nothing about graphics or GPUs, meshing, rasterization, or any of that advanced stuff (looong before I started learning Rust, let alone working on real graphics for Kovarust).
What I did know, however, was simple math - for each horizontal screen position, I raycast in the appropriate direction and drew a column inverse to the distance. While I was at it, I figured raycasting would make it easy to add non-Euclidean spaces (by way of portals), so I did that and ended up with a pretty neat terminal maze explorer.
(ok I cheated actually, I made something vaguely similar in a BASIC game-making app ages ago, but it was pretty janky)
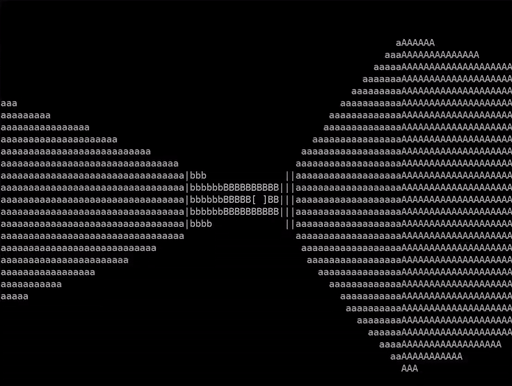
3D Engine
After doing the above 2D raycaster, I figured it might not actually be that hard to make something similar to real GPU rasterization in the terminal with basic trig (matrices were still a foreign concept to me). Halfway through the project I quickly threw full triangle rasterization out the window, but I was left with a true 3D environment and wireframe props.
I didn't really know what to do with this - the original idea was to publicize it as some sort of rendering engine, but without rasterization it'd be a bit hard to look at for long periods of time. So instead, I turned it into a demo for rotating stuff using trig functions in 2, 3, and because why not, 4 dimensions.
good luck decrypting my old C code tho lol
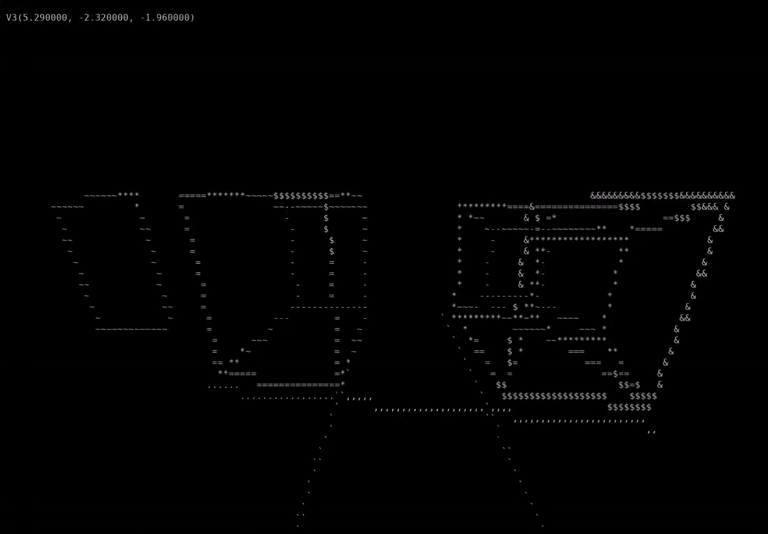